If you’re new to programming, Java is a great language to start with. It’s versatile, widely used in enterprise applications, web development, mobile apps, and more. Whether you’re building desktop applications or Android apps, Java provides a solid foundation java. In this guide, we’ll walk you through the process of building your first Java application from scratch.
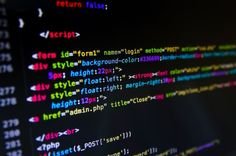
Prerequisites
Before we dive into the code, you’ll need a few things to get started:
- Java Development Kit (JDK): Java requires a set of tools for compiling and running programs. You can download the latest JDK from Oracle’s website or use an open-source version like OpenJDK.
- Text Editor or Integrated Development Environment (IDE): While you can use any text editor, IDEs like IntelliJ IDEA, Eclipse, or NetBeans make the process smoother with features like code completion and debugging.
- Basic Knowledge of Programming: Understanding basic programming concepts such as variables, loops, and conditionals will help you follow along with this tutorial.
Step 1: Set Up Your Development Environment
- Download and Install the JDK
- Go to Oracle’s website or AdoptOpenJDK to download the JDK.
- Follow the installation instructions specific to your operating system (Windows, macOS, or Linux).
- Install an IDE
- For beginners, IntelliJ IDEA is highly recommended because of its user-friendly interface. You can download the community edition for free from JetBrains.
- Alternatively, Eclipse is another popular choice for Java developers and can be downloaded from Eclipse Downloads.
- Verify Installation
- Open a terminal or command prompt and type
java -version
andjavac -version
. This will display the installed versions of the Java runtime and compiler. - If everything is installed correctly, you should see version details printed to the screen.
- Open a terminal or command prompt and type
Step 2: Create Your First Java Program
Now that your environment is set up, it’s time to create your first Java program. Follow these steps:
- Create a New Project
- In your IDE, create a new project. This can usually be done by selecting “New Project” from the IDE’s welcome screen.
- Name your project “FirstJavaApp” or something similar.
- Create a New Java Class
- In your project, create a new Java class. In IntelliJ IDEA, right-click on the
src
folder, select “New,” then choose “Java Class.” Name the classHelloWorld
to match the traditional first Java program.
- In your project, create a new Java class. In IntelliJ IDEA, right-click on the
- Write the Code Inside your
HelloWorld
class, type the following code:javaCopy codepublic class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Here’s what this code does:public class HelloWorld
: Declares a public class namedHelloWorld
.public static void main(String[] args)
: Defines themain
method, which is the entry point for Java applications.System.out.println("Hello, World!");
: Prints the text “Hello, World!” to the console.
Step 3: Compile and Run Your Program
- Compile the Program
- In most IDEs, running your program is as simple as clicking a “Run” button. IntelliJ IDEA will automatically compile your code when you hit the “Run” button.
- If you’re using the command line, navigate to the folder where your
HelloWorld.java
file is saved and run:Copy codejavac HelloWorld.java
This will generate a file calledHelloWorld.class
, which is the compiled bytecode.
- Run the Program
- To run the program, either use the “Run” button in your IDE or, from the command line, type:Copy code
java HelloWorld
- If everything is set up correctly, you should see the output:Copy code
Hello, World!
- To run the program, either use the “Run” button in your IDE or, from the command line, type:Copy code
Step 4: Understand the Code
Let’s break down the code and understand its components:
- Class Declaration (
public class HelloWorld
): In Java, all code must reside within a class. Thepublic
keyword indicates that this class is accessible from other classes. - Main Method (
public static void main(String[] args)
): This is the entry point of any Java application. TheString[] args
parameter allows you to pass command-line arguments to your program, but for this simple example, we don’t use it. - Printing to the Console (
System.out.println
): This is a method call that outputs text to the console.System.out
represents the standard output stream, andprintln
prints the message followed by a new line.
Step 5: Modify Your Program
To get familiar with Java, try modifying your program. For example, you can change the message to say something different, or add more statements.
javaCopy codepublic class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
System.out.println("Welcome to Java programming!");
}
}
After making changes, recompile and run the program as you did earlier. You’ll see the new output printed to the console.
Step 6: Explore More
Now that you’ve written your first Java program, you’re ready to explore more complex concepts. Here are a few suggestions for next steps:
- Variables and Data Types: Learn about different data types like
int
,double
,boolean
, andString
, and how to store values in variables. - Control Flow: Experiment with conditional statements (
if
,else
,switch
) and loops (for
,while
). - Methods: Learn how to write and use methods to organize your code.
- Object-Oriented Programming: Java is an object-oriented language, so it’s essential to learn about classes, objects, inheritance, and polymorphism.
Conclusion
Congratulations! You’ve just built your first Java application. This is just the beginning of your Java journey. As you explore more features and concepts, you’ll gain confidence and experience with this powerful language. Keep experimenting, coding, and building — the possibilities are endless with Java!
4o mini